If you are a developer looking to integrate AI functionality into your apps or web services, look no further than OpenAI’s API. This is one of the most advanced Artificial General Intelligence companies. They offer free AI consumer products and services, and their API is public for developers to integrate into their services.
Developers can use this API in a wide range of apps and services. These include creating images from prompts, translating languages, answering queries, and more. In this article, we will show you how to use OpenAI’s API in Python. Let us dive in!
Generating an API key
To start building projects with OpenAI’s API, you will need an OpenAI account and an API key. Here is how to get your OpenAI key and account:
- Visit the OpenAI Platform and log in with your account. If you don’t have an account, click “Sign Up” and follow the instructions to create one.
- Verify your email by entering your details, or continue with Google or Microsoft authentication as a sign-in.
- After verifying your email, you will be asked to verify your mobile number.
- Once you verify your mobile number, you will be redirected to the OpenAI Platform.
- Click on your profile icon in the upper-right corner of the platform.
- Then, click on the “View API key.”
- From there, you can create a new secret key.
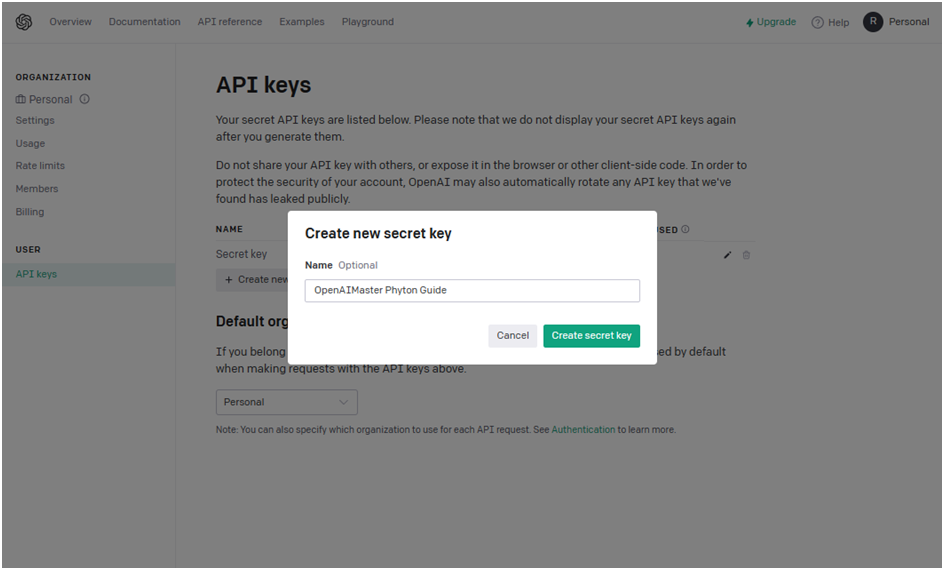
- Click on “Create a Secret key” and give it a name.
- Copy the Secret key to a safe location since you cannot view it again unless you create a new Secret Key.
- Save the key and start creating your OpenAI project.
Installing the OpenAI Python Library
To set up your developer environment, you will need a code editor of your choice. I recommend Microsoft’s Visual Studio.
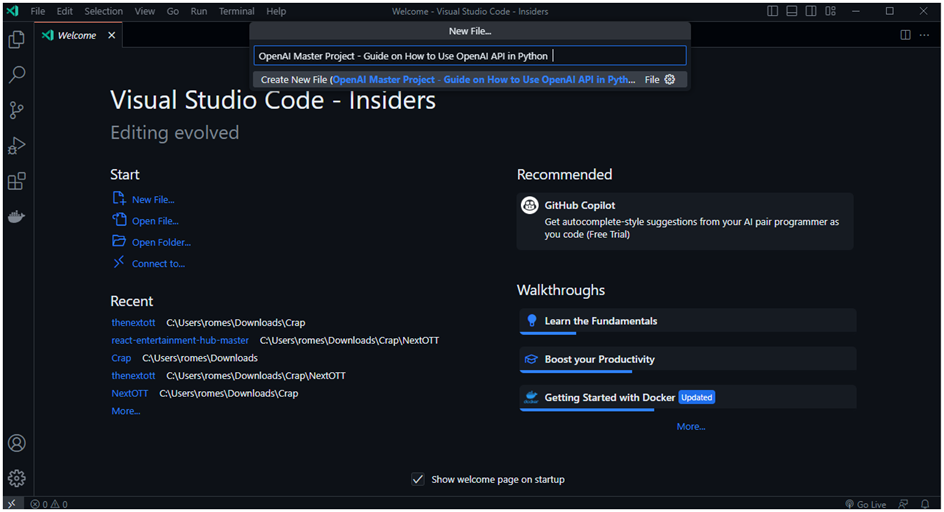
- Download, and Install the Python Interpreter on your Code editor.
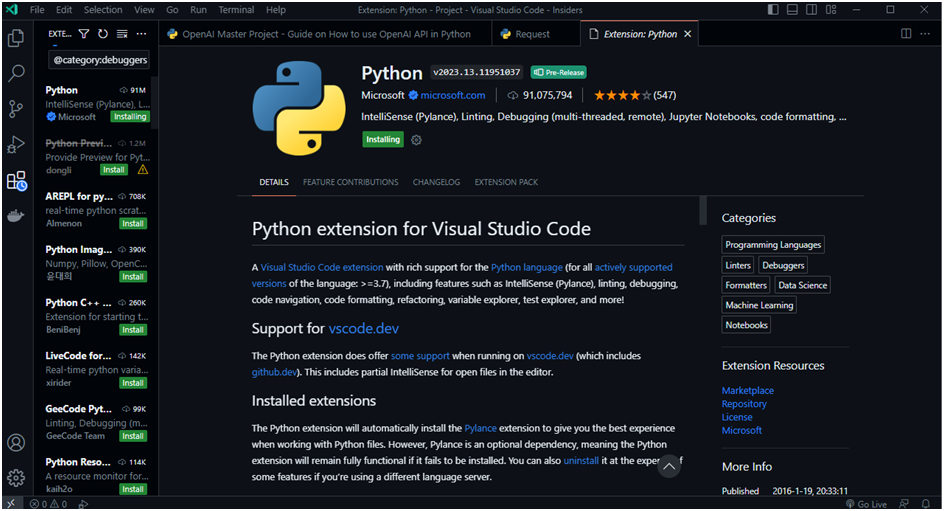
- Create a New Project (hotkey: Ctrl +Alt + Windows + N) and Save the project locally.
- After that, we need to Install the OpenAI Python Library, for which you need to run this command in Terminal or Command Prompt.
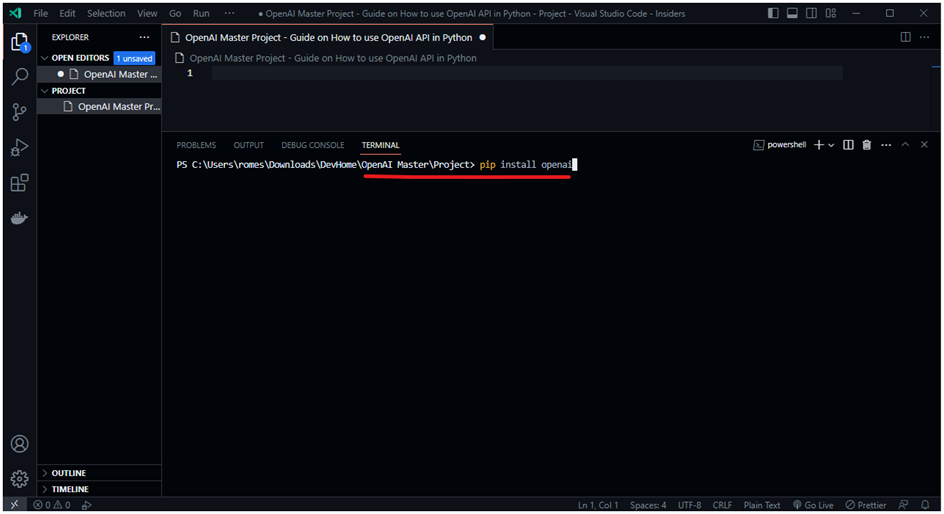
pip install openai
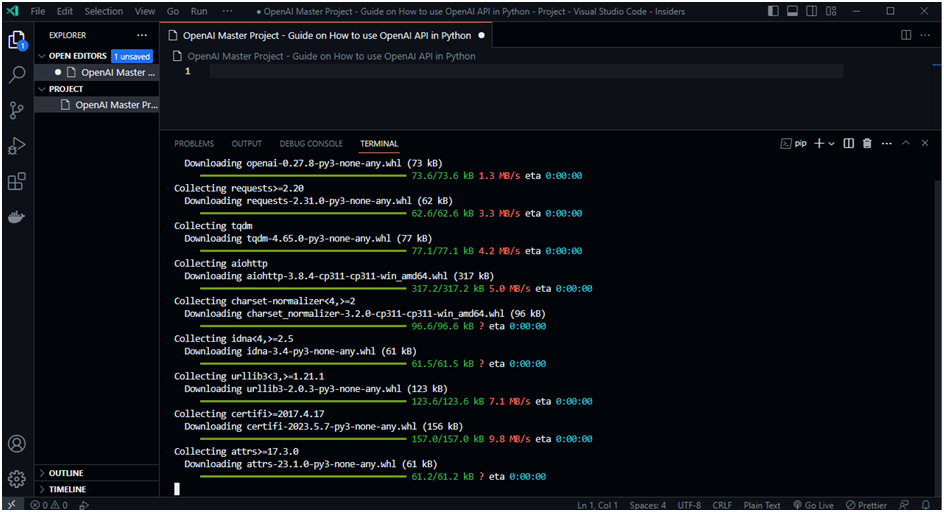
- After executing it, Now Update the OpenAI Python Library by executing. >python.exe -m pip install –upgrade pip
- Now, Once you have Installed the OpenAI Python Library, you can start integrating your API key into this code
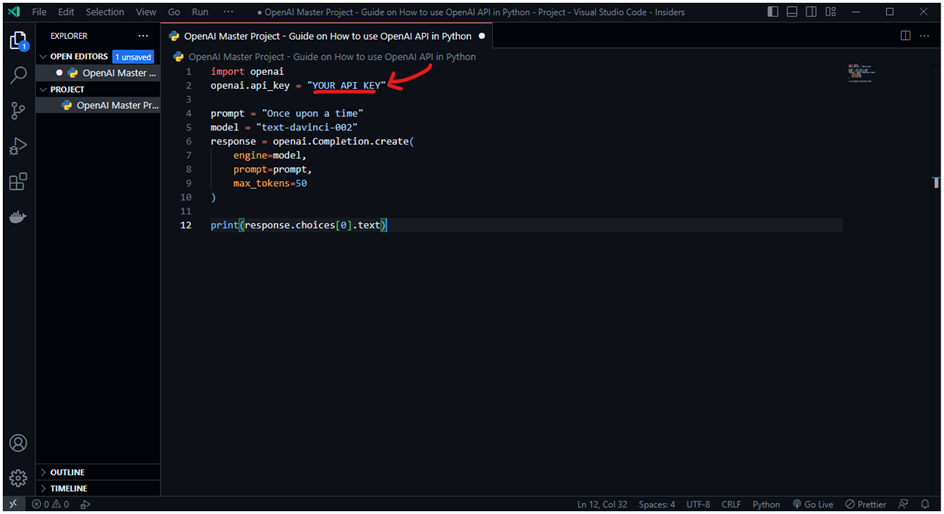
import openai
openai.api_key = “YOUR_API_KEY”
prompt = “Once upon a time”
model = “text-davinci-002”
response = openai.Completion.create(
engine=model,
prompt=prompt,
max_tokens=50
)
print(response.choices[0].text)
Note: You do need to modify the Mode (openai.Completion.create()), Model (text-davinci-002), and also check the maximum number of tokens you want to generate (max_tokens=50). Here are some basic parameters to learn:
- When you request with openai.Completion.create, there are several parameters that you can adjust to get the expected response: *
- engine: It is used for completions, models like text-davinci-002 and text-curie-002.
- prompt: It starts the text generation upon receiving the prompt.
- max_tokens: It is the maximum length allowed for users to enter characters, and these are the tokens used during API calls.
- temperature: It controls how creative you want the AI model to be and ranges from 0 to 10.
- top_p: It uses nucleus sampling and limits the model’s output to the most probable tokens.
How to Authenticate Your OpenAI Key
To authenticate your API key, you can directly do so within your code by importing openai. Use the Developer-Environment with the following command:
- Unix: export OPENAI_API_KEY=’your-api-key’
- Windows: setx OPENAI_API_KEY “your-api-key”
Requesting OpenAI API
Once you have set up everything with OpenAI in Python, you can start making API calls. To do this, use the following code to request OpenAI to “Write a poem about a cat”:
import openai
client = openai.Client()
response = client.create("Write a poem about a cat", prompt="Write a poem about a cat")
print(response)
Another way to interact with the OpenAI API is by using the OpenAI Python Library with gpt-3.5-turbo mode. Here’s an example:
chat_completion = openai.ChatCompletion.create(
messages=[{"role": "user", "content": "Hello world"}]
)
print(chat_completion.choices[0].message.content)
You can find the OpenAI API Python Library and other useful codes, including usages, Microsoft Azure endpoint, command line, chat completion, embedding, DALL-E, Whisper, and more by visiting the OpenAI API Python Library.
Developers are advised to experiment and learn prompt engineering to design more specific, niche, and efficient applications. OpenAI provides all the necessary documentation, references, resources, and support through its community. Remember to check out the OpenAI playground for codes and examples to learn and test many different things.
That is how to use the OpenAI API in Python, which seems straightforward. YouTube has several project lessons, primarily via FreeCodeCamp. Check the GitHub repository for excellent practices and resource utilization to get inspiration. Hopefully, this guide remains helpful, and you have successfully used the OpenAI API in Python.